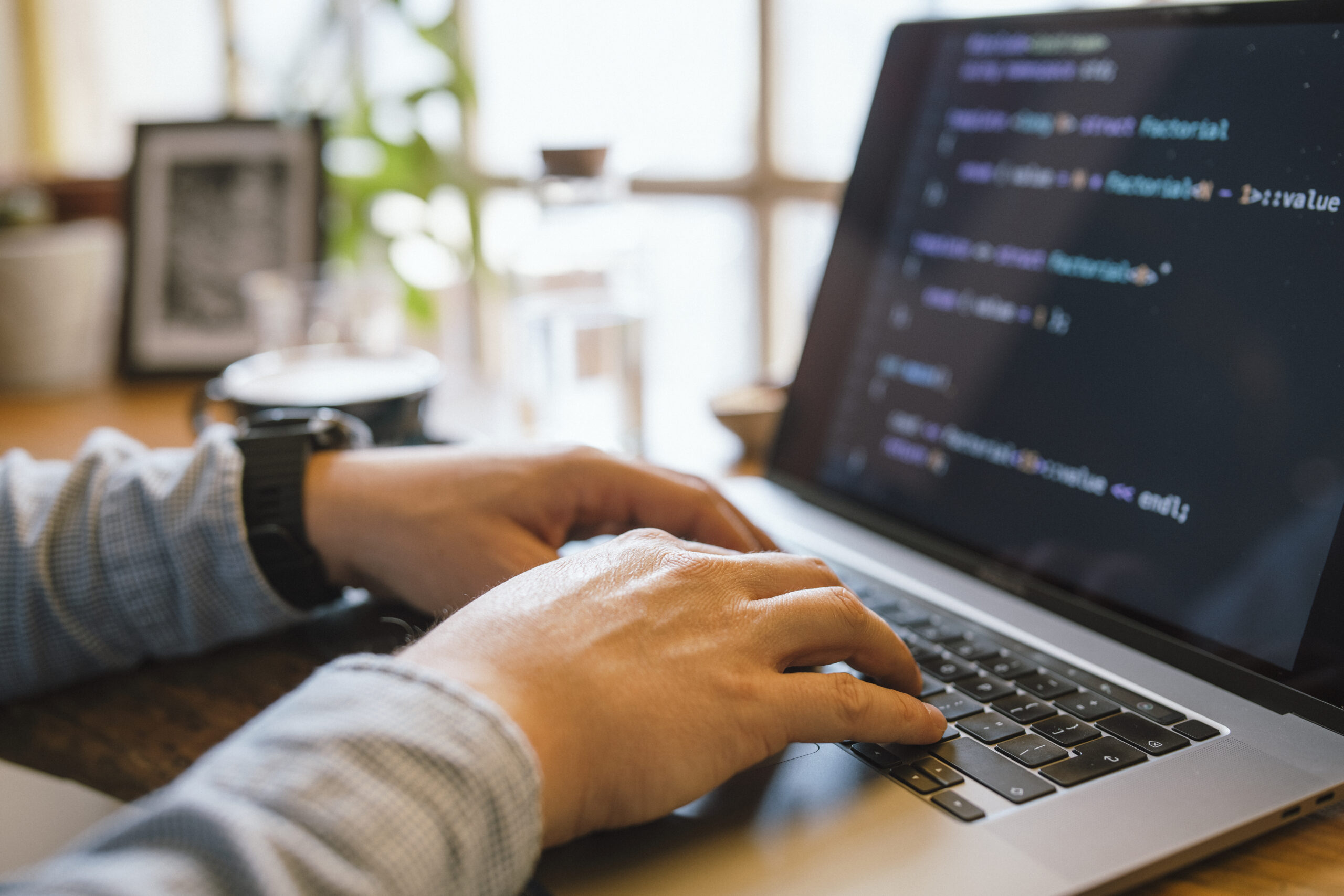
Debugging is one of the most vital — nonetheless frequently disregarded — techniques in a developer’s toolkit. It's not nearly fixing broken code; it’s about comprehension how and why points go Completely wrong, and Discovering to think methodically to solve problems efficiently. Whether or not you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of aggravation and significantly enhance your productivity. Here are several strategies that will help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest approaches developers can elevate their debugging abilities is by mastering the tools they use everyday. When producing code is one particular Portion of development, recognizing tips on how to communicate with it successfully during execution is Similarly crucial. Contemporary enhancement environments appear equipped with impressive debugging capabilities — but many builders only scratch the surface area of what these equipment can perform.
Get, for instance, an Built-in Growth Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments help you set breakpoints, inspect the worth of variables at runtime, phase by means of code line by line, and also modify code within the fly. When employed appropriately, they let you notice precisely how your code behaves throughout execution, which is a must have for tracking down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for entrance-close developers. They assist you to inspect the DOM, check community requests, view true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can convert frustrating UI troubles into workable tasks.
For backend or program-amount builders, resources like GDB (GNU Debugger), Valgrind, or LLDB offer you deep control above jogging procedures and memory management. Finding out these applications may have a steeper Understanding curve but pays off when debugging effectiveness challenges, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into at ease with version Manage techniques like Git to be aware of code record, find the exact moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications usually means going beyond default settings and shortcuts — it’s about creating an intimate knowledge of your improvement surroundings so that when issues arise, you’re not lost in the dark. The better you know your tools, the greater time you could expend resolving the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the challenge
The most vital — and often ignored — steps in effective debugging is reproducing the condition. In advance of leaping in to the code or creating guesses, builders will need to make a constant environment or state of affairs wherever the bug reliably appears. Without reproducibility, correcting a bug gets a recreation of opportunity, normally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as possible. Check with inquiries like: What actions brought about the issue? Which ecosystem was it in — growth, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you've, the a lot easier it gets to isolate the exact problems below which the bug takes place.
When you’ve gathered sufficient facts, make an effort to recreate the condition in your local natural environment. This could indicate inputting the same knowledge, simulating equivalent person interactions, or mimicking program states. If The difficulty appears intermittently, take into account crafting automated assessments that replicate the sting instances or condition transitions associated. These tests not merely assistance expose the issue and also prevent regressions Later on.
From time to time, the issue could be natural environment-specific — it might transpire only on sure operating techniques, browsers, or underneath individual configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t merely a move — it’s a mindset. It demands persistence, observation, plus a methodical tactic. But when you finally can continuously recreate the bug, you're currently halfway to fixing it. Having a reproducible scenario, You can utilize your debugging equipment a lot more properly, examination likely fixes safely and securely, and converse far more Plainly using your crew or end users. It turns an abstract grievance into a concrete challenge — Which’s wherever builders thrive.
Go through and Realize the Error Messages
Error messages will often be the most valuable clues a developer has when a little something goes Completely wrong. In lieu of observing them as discouraging interruptions, builders must discover to treat mistake messages as direct communications in the system. They normally inform you what exactly occurred, where it transpired, and often even why it occurred — if you know the way to interpret them.
Start out by reading through the message diligently and in full. Lots of developers, especially when underneath time stress, look at the primary line and instantly get started generating assumptions. But deeper from the error stack or logs may well lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — go through and understand them 1st.
Break the error down into parts. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line range? What module or function activated it? These questions can information your investigation and point you toward the liable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re working with. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Understanding to acknowledge these can drastically accelerate your debugging system.
Some mistakes are obscure or generic, As well as in those circumstances, it’s very important to examine the context during which the mistake happened. Check connected log entries, enter values, and up to date adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede more substantial challenges and provide hints about possible bugs.
Eventually, mistake messages are certainly not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and turn into a more efficient and confident developer.
Use Logging Wisely
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When applied correctly, it offers authentic-time insights into how an software behaves, serving to you have an understanding of what’s going on underneath the hood while not having to pause execution or action from the code line by line.
A fantastic logging tactic commences with being aware of what to log and at what stage. Frequent logging amounts contain DEBUG, Information, WARN, Mistake, and Deadly. Use DEBUG for thorough diagnostic data in the course of advancement, Information for general situations (like thriving start out-ups), WARN for possible issues that don’t crack the appliance, ERROR for precise challenges, and Deadly when the system can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure critical messages and slow down your procedure. Center on crucial occasions, point out alterations, input/output values, and significant selection details with your code.
Format your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re especially worthwhile in production environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. By using a well-imagined-out logging tactic, you are able to decrease the time it's going to take to spot concerns, get further visibility into your applications, and Enhance the Over-all maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not merely a technical activity—it's a kind of investigation. To proficiently detect and repair bugs, developers have to tactic the procedure similar to a detective solving a mystery. This attitude will help stop working advanced challenges into workable parts and adhere to clues logically to uncover the root result in.
Start off by collecting proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, collect just as much relevant information as you can with out jumping to conclusions. Use logs, test cases, and person experiences to piece jointly a transparent image of what’s taking place.
Subsequent, type hypotheses. Ask yourself: What could be producing this actions? Have any improvements just lately been created for the codebase? Has this problem happened right before underneath related situations? The goal would be to slender down options and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation within a controlled natural environment. In case you suspect a specific functionality or ingredient, isolate it and verify if The difficulty persists. Like a detective conducting interviews, ask your code issues and Allow the effects direct you closer to the reality.
Spend shut focus to small facts. Bugs usually disguise while in the least envisioned areas—similar to a missing semicolon, an off-by-a person error, or a race issue. Be thorough and individual, resisting the urge to patch the issue with no fully comprehension it. Temporary fixes may possibly hide the true problem, only for it to resurface afterwards.
Finally, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging approach can help you save time for potential difficulties and help Other folks have an understanding of your reasoning.
By pondering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become more effective at uncovering hidden difficulties in complex methods.
Publish Checks
Crafting tests is one of the best strategies to help your debugging skills and General advancement effectiveness. Assessments not simply assistance capture bugs early but also serve as a safety net that gives you self-confidence when producing alterations to the codebase. A very well-analyzed software is simpler to debug as it means that you can pinpoint accurately where by and when a dilemma takes place.
Get started with device assessments, which center on particular person features or modules. These modest, isolated assessments can swiftly expose irrespective of whether a selected bit of logic is Doing work as anticipated. Whenever a check fails, you instantly know where to look, noticeably lessening enough time put in debugging. Unit tests are Primarily handy for catching regression bugs—troubles that reappear right after previously being fastened.
Following, integrate integration checks and conclusion-to-conclude exams into your workflow. These help be sure that a variety of elements of your software get the job done with each other smoothly. They’re specially beneficial for catching bugs that happen in elaborate programs with numerous factors or companies interacting. If some thing breaks, your checks can let you know which part of the pipeline failed and under what ailments.
Creating checks also forces you to Assume critically about your code. To check a function thoroughly, you will need to understand its inputs, predicted outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continually, you can target correcting the bug and view your examination go when the issue is settled. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from the discouraging guessing game into a structured and predictable approach—serving to you capture more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to be immersed in the situation—gazing your monitor for hours, attempting Remedy soon after Option. But One of the more underrated debugging tools is simply stepping absent. Taking breaks helps you reset your mind, decrease aggravation, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive exhaustion sets in. You might start overlooking obvious errors or misreading code that you choose to wrote just several hours earlier. In this point out, your Mind gets considerably less productive at difficulty-solving. A short wander, a espresso split, or perhaps switching to a different endeavor for ten–15 minutes can refresh your concentrate. Numerous builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious perform within the history.
Breaks also enable avert burnout, Specifically during for a longer period debugging periods. Sitting before a display, mentally trapped, is not simply unproductive but additionally draining. Stepping absent means that you can return with renewed Vitality and a clearer way of thinking. You could possibly all of a sudden see a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, an excellent general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It could really feel counterintuitive, In particular under restricted deadlines, but it in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, taking breaks is just not an indication of weakness—it’s a wise strategy. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a psychological puzzle, and relaxation is an element of solving it.
Understand From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural issue, each one can educate you anything important if you take some time to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of crucial inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved practices like device tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or being familiar with and assist you Establish much better coding patterns going ahead.
Documenting bugs can even be a fantastic routine. Hold a developer journal or sustain a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you figured out. After some time, you’ll begin to see patterns—recurring problems or common issues—you could proactively prevent.
In crew environments, sharing Everything you've discovered from the bug with the peers might be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a check here brief publish-up, or a quick knowledge-sharing session, serving to Other folks avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. In lieu of dreading bugs, you’ll commence appreciating them as essential portions of your improvement journey. In fact, several of the best builders are not the ones who generate best code, but those who continually learn from their problems.
Eventually, Every single bug you take care of adds a different layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Conclusion
Bettering your debugging techniques requires time, follow, and tolerance — but the payoff is big. It would make you a far more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.